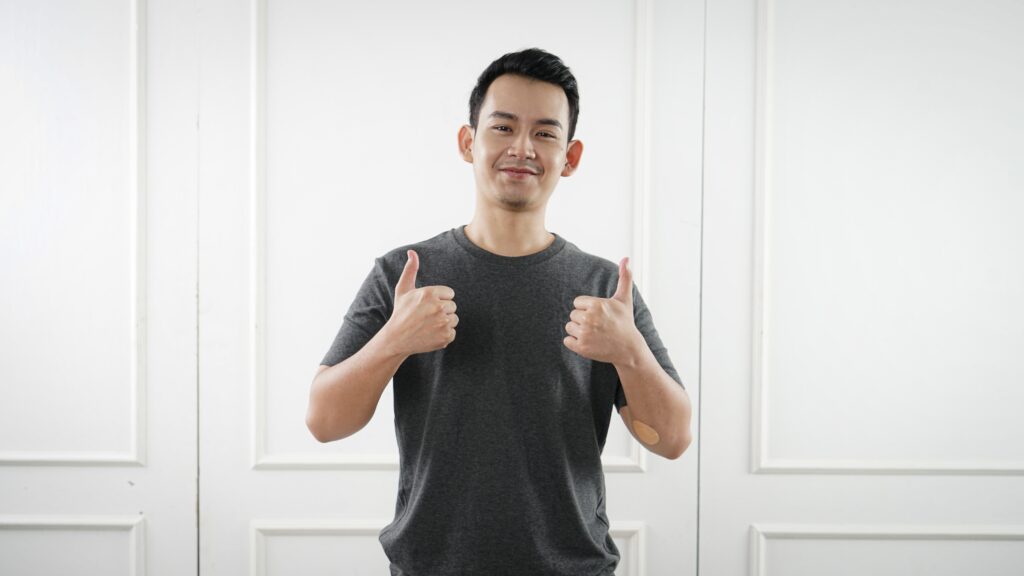
Once we have successfully made a request to the maintenanceman.nl API Server, we will get a http response with a response body containing the results of our query. For this example we will dissect the response for the request made in the previous post: ‘Setting up your first API request’. It is highly recommended to read that post before continuing here.
Response status
The first line of feedback comes from the http status code in the response. This follows the standard http response code specifications, where 200 indicates a successful request, codes in the 400 range indicate problems in the request (authorization issues, invalid input, etc.) and 500 indicates a server error, which should of course not occur. You should only process responses with the 200 status code.
Response body
Once you get a response with a 200 or 400 range status code, it will also contain a response body in application/json format. The JSON object in the response will at least have the following fields:
- error:
Boolean value indicating if a fatal error occured while processing the request. This is complimentary to the status code. - message:
String value, containing a general message on the the response. Usually error descriptions or general notions of a successful request. For debugging this field is important to check. - content:
JSON object containing the actual result. This is empty for failed requests.
The content object
For successful requests, the above mentioned content field will contain a JSON object with two fields named ‘errors‘ and ‘result‘. The errors field will contain an array of strings, each indicating a non fatal error that occurred while processing your request. If this array is not empty it is important to scheck its contents as this may indicate inconsistencies in your input and possibly unexpected output that may not be obvious at first glance. Finally, the result field will of course contain the actual outcome of your query in the form of an array of JSON objects, each one representing a future execution of the schedule items as specified in the request body.
Result array
Each object in the result array will consist of the following fields:
- id:
This is the schedule item id as provided in the request body. - name:
This is the schedule item description as provided in the request body. - value:
This is the value for the measure of asset usage at which the schedule item execution should take place. (For our example truck, this is if course the mileage in kilometers). Please note that for schedule items that are overdue as per provided data in the request, this value will be equal to the provided ‘currentUnits‘ value in the request as the schedule item is immediately due and cannot be executed before present time. Please note that the original value at which the schedule item execution should have taken place, will still be indicated in the ‘dueAt’ field if the due date was usage based. If the due date is calendar based, the actual due date will be provided in the ‘dueDate’ field. - date:
This field contains the execution date corresponding to the value field, formatted as per provided format in the request body. - rawDate:
Same value as the date field, but formatted as Unix Timestamp. - year:
Calendar year of execution date. - dueAt:
Only provided for overdue schedule item executions that are due based on the measure of asset usage. This reflects the actual value at which the schedule item was due. - dueDate:
Only provided for overdue schedule item executions that are due based on a calandar date. This reflects the actual date at which the schedule item was over due.
Going back to our example truck. The response body from our request would look like:
{
"error": false,
"message": "See results in content.",
"content":
{
"errors": [ ],
"result":
[{
"id": "INT",
"name": "Intermediate service",
"value": 98500,
"date": "2023-11-16",
"rawDate": 1700089200,
"year": 2023,
"dueAt": 0, "dueDate": ""
},{
"id": "OIL",
"name": "Oil change",
"value": 118500,
"date": "2024-5-16",
"rawDate": 1715810400,
"year": 2024,
"dueAt": 0, "dueDate": ""
},{
"id": "INT",
"name": "Intermediate service",
"value": 133509,
"date": "2024-9-29",
"rawDate": 1700089200,
"year": 2024,
"dueAt": 0, "dueDate": ""
}]
}
}
Please note that the result array in the above example only contains three items, but in reality the array will of course be much longer.
As you will see, our example truck will be due for its next intermediate service by November 16th 2023 and for an oil change by may 16th, 2024, etc, etc, etc.
Alternative response body
Instead of the response body as described above, it is also possible to get a simplified response in the form of a two dimensional array containing only the values of the result field. This is done by changing the value “JSON” in the “resultType” field of the request body to any other value.
The resulting array will only contain the columns ‘id‘, ‘name‘, ‘value‘, ‘date‘, ‘year‘, ‘dueAt‘ and ‘dueDate‘ and will be directly provided in the ‘content‘ field of the response body. The array does not include header names. This methods is not recommended as it will also omit the errors field, making possible flaws in the input invisible.