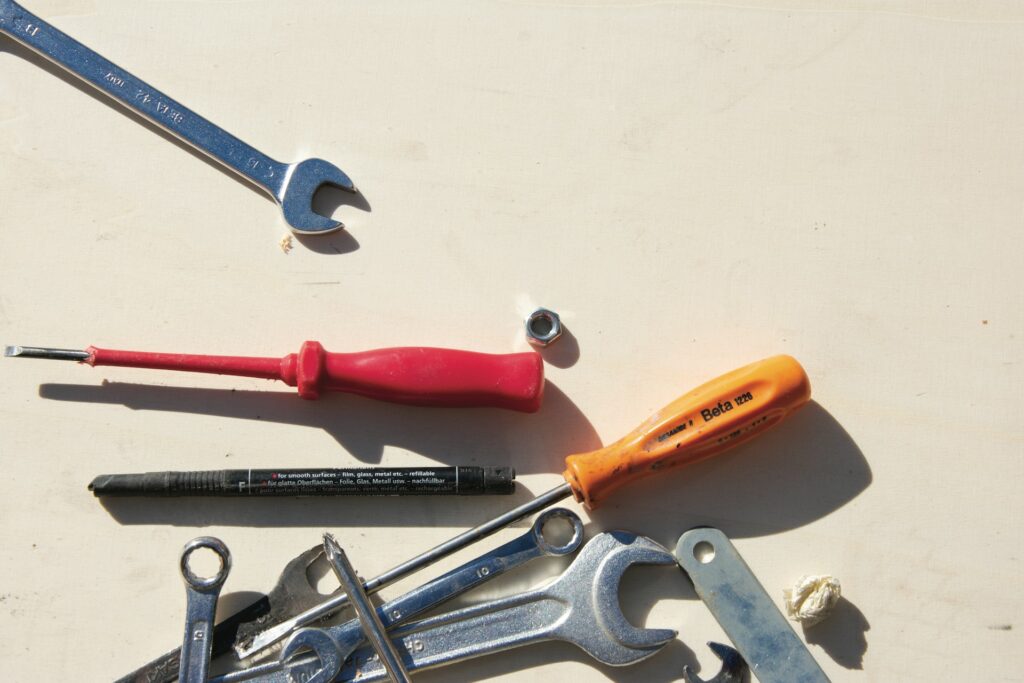
A proper http request should have valid request headers and a valid request body. Please note that this guide assumes that you already know your way around http requests and how to make them in the programming environment you are working with. If that is not the case, you might want to study that before going further. If you are not a programmer, but do want to use the API in a low code environment, you may like the guide for using maintenanceman.nl API from a spreadsheet, but please note that this is not a safe method for use in production environments as it does not provide any way to properly protect your secret API Key from being shared with others.
That being said, let’s now dive into the details of your http request.
Request headers:
The request headers to be included with every request are quite simple. The only headers required are:
- X-RapidAPI-Key:
This is your personal API Key. This will be used to identify and properly bill your account.
- X-RapidAPI-Host:
The value for this is always ‘maintenanceman-nl-asset-management-api.p.rapidapi.com’. This is used to properly redirect your request to the maintenanceman.nl servers.
- x-rapidapi-ua:
The value for this is always ‘RapidAPI-Playground’. This is an internal requirement specific for rapidAPI.
- content-type:
this is always ‘application/json’ and used to set the type of request body. If this is not properly set, the response will be in html format instead of a JSON object and will not contain relevant data.
Request method:
As your request will contain a JSON body, the proper request method is POST. Any other request methods will fail.
Request URL:
Any http request of course requires a proper URL to address the request to. This is always ‘https://maintenanceman-nl-asset-management-api.p.rapidapi.com‘ followed by the endpoint as specified in the API documentation. As there is currently just one endpoint, the URL will always be ‘https://maintenanceman-nl-asset-management-api.p.rapidapi.com/schedule‘.
Request body:
Now we are past the formalities, let’s dive into the request body. This is where the actual magic happens and where you specify the details of your request. As mentioned before, the request body will contain a single JSON object, that contains all relevant data for the API to work with. For this example we will assume that you have a truck that needs maintenance based on the mileage it has run.
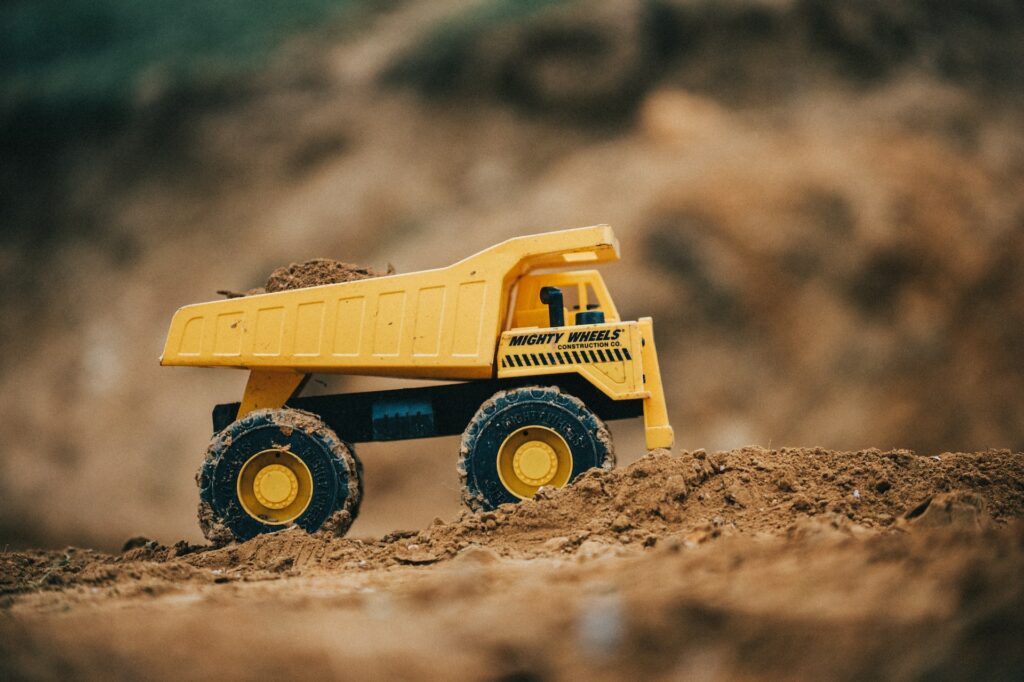
First we will setup the general properties of the truck with the parameters unitsPerDay, currentDate and currentUnits. The unitsPerDay parameter specifies the expected average number of units per day for an unspecified measure of asset usage. This sounds a bit complicated, but in our example this is just the average distance the truck is expected to travel per day. Let’s assume that we expect the truck to drive 40.000 kilometres per year. In that case the average number of kilometers per day will be 40.000 / 365 = 109,6 kilometers per day. It is recommended to use an integer value, so we will round the unitsPerDay value to 110 km/day. Obviously, this parameter will be one of the main parameters to play with when simulating different scenarios.
Please note that the API does not actually care about the unit of measure and this will not be specified in the request body. Instead of kilometres, you could use the value of any unit of measure that is relevant to your asset, such as running hours, miles, oscillations, trips to the moon or whichever other unit of measure you could think of. For this example however, we will stick to kilometers.
Now that we understand the unitsPerDay parameter, the other two become self explanatory. The currentUnits parameter of course specifies the actual current value for asset usage and thus the actual mileage of the truck in our example in kilometres and the currentDate value is the actual date that this value was registered. Of course the term ‘current’ can be read as ‘last known’ here and does not need to be the exact current value if that is not known. For our example we will assume that the truck has already run 90.000 kilometres on August 31st, 2023.
Besides the aforementioned parameters, there are four other general parameters to specify, which are the dateZero, resultType, dateFormat and period parameters. For details on these parameters, please see the official API documentation. For the period parameter, it is important to know that this specifies the total period to cover in the results, specified in days. This value is automatically limited to the equivalent of 50 years in days, but for performance reasons it is recommended to use a shorter period.
For this example we will set the period to 3.653 days, which equals a time span of 10 years. For accuracy it is recommended to keep this period slightly longer than the period you wish to examine.
Also we will set the responseType to JSON and the dateFormat to Y-m-d
So far our request body looks as shown below.
{
"unitsPerDay":110,
"currentUnits":90000,
"currentDate":"2023-08-31",
"dateZero":"2010-01-01",
"period":3653,
"responseType":"JSON",
"dateFormat":"Y-m-d"
}
Next we will specify the maintenance schedule to be simulated and the current state of maintenance of our asset. This is all done inside the schedule parameter, which will contain an array of JSON objects, each specifying a rule for the maintenance schedule that is applicable for our asset.
Let’s assume that our example truck will need an oil change at every 20.000 kilometers. To have this reflected in the request body we will add a new object to the schedule array with the following fields.
{
"id": "OIL",
"name":"Oil change"
"interval":{units: 20000},
"lastExecutedDate":"2023-07-10",
"lastExecutedValue":83500
}
The id field is mandatory and is used to make reference to this specific schedule rule as we will see later. For readability, it is recommended to use a value that is easy to recognize. Also its value should of course be unique in the array and must be made up from alphanumeric characters.
The name field is optional and can be used for a more reader friendly description of the rule.
You will notice that the interval field is also a JSON object, which, for now, will contain only one field named ‘units’, which is set to 20.000 kilometers. For more complex use cases, more fields will be specified, but we will get to that later.
Of course we also have to specify when the last known execution of this schedule item took place, which is done in the lastExecutedDate and lastExecutedValue fields. For this example we will assume that the last oil change took place on July 10th, 2023 at a mileage of 83500 kilometers.
Now the request body will look as follows:
{
"unitsPerDay":110,
"currentUnits":90000,
"currentDate":"2023-08-31",
"dateZero":"2010-01-01",
"period":3653,
"responseType":"JSON",
"dateFormat":"Y-m-d"
"schedule":[{
"id": "OIL",
"name":"Oil change"
"interval":{units: 20000},
"lastExecutedDate":"2023-07-10",
"lastExecutedValue":83500
}]
}
This is the most basic, functional request body that will produce a valid response, but of course our truck will need more than just oil changes. Let’s add another rule to the maintenance schedule to make it a bit more realistic.
We will assume that our truck will require an intermediate service every 35.000 kilometers, which includes an oil change. To do this, we will add a new object to the schedule array that looks like:
{
"id": "INT",
"name":"Intermediate service"
"interval":{units: 35000},
"lastExecutedDate":"2021-12-10",
"lastExecutedValue":63500
"willReset":"OIL"
}
As you will see, this schedule item is not much different from the previous one, except for the willReset field. This can contain a string value with one or more schedule item id‘s, separated by commas. If this field is set, the referenced schedule items will restart counting their interval when the current schedule item is executed.
In our example, the intermediate service will include an oil change, so the oil change interval can start counting from zero when the intermediate service is executed.
The full request body now looks like:
{
"unitsPerDay":110,
"currentUnits":90000,
"currentDate":"2023-08-31",
"dateZero":"2010-01-01",
"period":3653,
"responseType":"JSON",
"dateFormat":"Y-m-d"
"schedule":[{
"id": "OIL",
"name":"Oil change"
"interval":{units: 20000},
"lastExecutedDate":"2023-07-10",
"lastExecutedValue":83500
},{
"id": "INT",
"name":"Intermediate service"
"interval":{units: 35000},
"lastExecutedDate":"2021-12-10",
"lastExecutedValue":63500
"willReset":"OIL"
}]
}
Following this logic, it is possible to endlessly extend and complicate the schedule object, however, it is recommendable to carefully evaluate the schedule you specify to make sure that it makes sense in order to prevent unexpected outcomes.
Finally, it is also possible to define a hard time limit within the interval objects, complimentary to the ‘units’ field, by adding an orDays field to the interval object.
Imagine that you would like to make sure that the oil change on our example truck is executed at least once a year, you could add the orDays field with a value of 365. If, for some reason, our truck will drive less than 20.000 kilometers per year, the oil change will still be initiated 365 days after the previous oil change.
With that addition, the request body now looks as follows:
{
"unitsPerDay":110,
"currentUnits":90000,
"currentDate":"2023-08-31",
"dateZero":"2010-01-01",
"period":3653,
"responseType":"JSON",
"dateFormat":"Y-m-d"
"schedule":[{
"id": "OIL",
"name":"Oil change"
"interval":
{
units: 20000,
orDays: 365
},
"lastExecutedDate":"2023-07-10",
"lastExecutedValue":83500
},{
"id": "INT",
"name":"Intermediate service"
"interval":{units: 35000},
"lastExecutedDate":"2021-12-10",
"lastExecutedValue":63500
"willReset":"OIL"
}]
}
The caveat with this is that time measurement in the maintenanceman.nl API is exclusively done in days, which makes it impossible to account for leap years and different lengths of months. If this is a problem for you, the best solution is to define a year as 365,25 days and a month as 30,438 days, but this will remain kind of a dirty workaround and will still result in slight time shifting over longer periods.
In summary, a http POST request containing the above mentioned headers and request body, addressed to the correct endpoint should result in a response that contains the dates and mileages at which our example truck will be due for an oil change or intermediate service, for the next 10 years. In the next post we will see how to interpret this response.
Please note that this example does not cover the full capability of the API. Please refer to the official documentation to get a full overview of all possible schedule parameters to be included.